Get the Python os Current Directory with os.getcwd(). Learn how to navigate and change the working directory easily.
Python os Current Directory
Retrieving Current Directory
Python os Current Directory, To retrieve the current directory in Python using the os
module, utilize the os.getcwd()
method. This function returns a string representing the current working directory.
This code snippet demonstrates how to obtain the current directory:
python import os current_directory = os.getcwd() print(“Current Directory:”, current_directory)
Changing Current Directory
To change the current directory in Python, you can use the os.chdir()
method. This function allows you to set a new path as the current working directory.
Here’s an example illustrating how to switch the current directory using path objects.
python import os new_directory = “/path/to/new/directory” os.chdir(new_directory) print(“Current Directory changed to:”, os.getcwd())
Listing Files in Current Directory
You can list all files and directories within the current directory using os.listdir()
. This function returns a list of all entries in the directory.
Consider this code snippet to display all files and directories in the current working directory:
python import os files_in_directory = os.listdir() for item in files_in_directory: print(item)

Accessing os Current Directory in Python
Retrieving Current Directory
To access the current directory in Python, use the os module. The os.getcwd() function returns the current working directory as a string.
Listing Directory Contents
You can list all files and directories in the current directory using os.listdir(). This function returns a list of all the files and directories in the specified path.
Changing the Current Directory
To change the current directory, utilize os.chdir() by passing the path of the desired directory as an argument. This function changes the current working directory to the specified path.
Checking if a Path is a Directory or File
Use os.path.isdir() to check if a given path is a directory. Similarly, os.path.isfile() determines if a given path is a file.
Handling Paths Safely
When dealing with paths, it’s crucial to ensure their validity. os.path.exists() checks whether a path exists, while os.path.abspath() returns the absolute path of a specified location.
Changing Current Directory in Python
Using os.chdir
To change the current directory in Python, you can utilize the os.chdir
method. This function allows you to specify the path of the directory you want to switch to. For example, by calling os.chdir('/path/to/directory')
, you can navigate to a different directory within your Python script.
Checking the Current Directory
Before changing the current directory, it’s essential to verify the current working directory. You can achieve this by using the os.getcwd()
function. This method returns the current working directory as a string, enabling you to confirm the directory before making any changes.
Best Practices
When changing directories in Python, ensure that the specified path is accurate and exists. Incorrect paths can lead to errors and unexpected behavior in your script. Remember to handle exceptions appropriately when dealing with file operations to prevent crashes and improve the robustness of your code.

Exploring os.getcwd() Method in Python
Overview
The os.getcwd() method in Python is a useful tool for obtaining the current working directory of a Python script. By calling this method, you can retrieve the path of the directory where your script is currently executing.
When you run a Python script, it operates within a specific directory on your file system. Understanding and being able to access this directory can be crucial for tasks involving file operations, such as reading or writing files.
Implementation
To utilize os.getcwd() and obtain the directory path, you simply need to import the os module in your Python script. Once imported, you can call the getcwd() method using the following syntax:
python import os current_directory = os.getcwd() print(“Current Working Directory:”, current_directory)
Benefits
- Convenience: Easily access the current working directory without manually tracking it.
- Portability: Ensures that your script can dynamically adapt to different working directories.
Path.cwd() Method for Directory
Obtaining Current Working Directory
The Path.cwd() method in Python is used to retrieve the current working directory. This method returns a Path object representing the current directory path.
Using this method eliminates the need to import the os module explicitly, as it is part of the pathlib module in Python.
Syntax and Implementation
To use the Path.cwd() method, you simply call it without any arguments. Here’s a simple example:
python from pathlib import Path
current_directory = Path.cwd() print(“Current Directory:”, current_directory)
Benefits of Using Path.cwd()
- Simplifies code: By directly accessing the current directory without importing the os module separately.
- Enhances readability: The use of Path objects makes the code more understandable and clean.
Example Use Case
Consider a scenario where you need to create a new file in the current working directory. By utilizing Path.cwd(), you can easily obtain the path and proceed with file operations seamlessly.
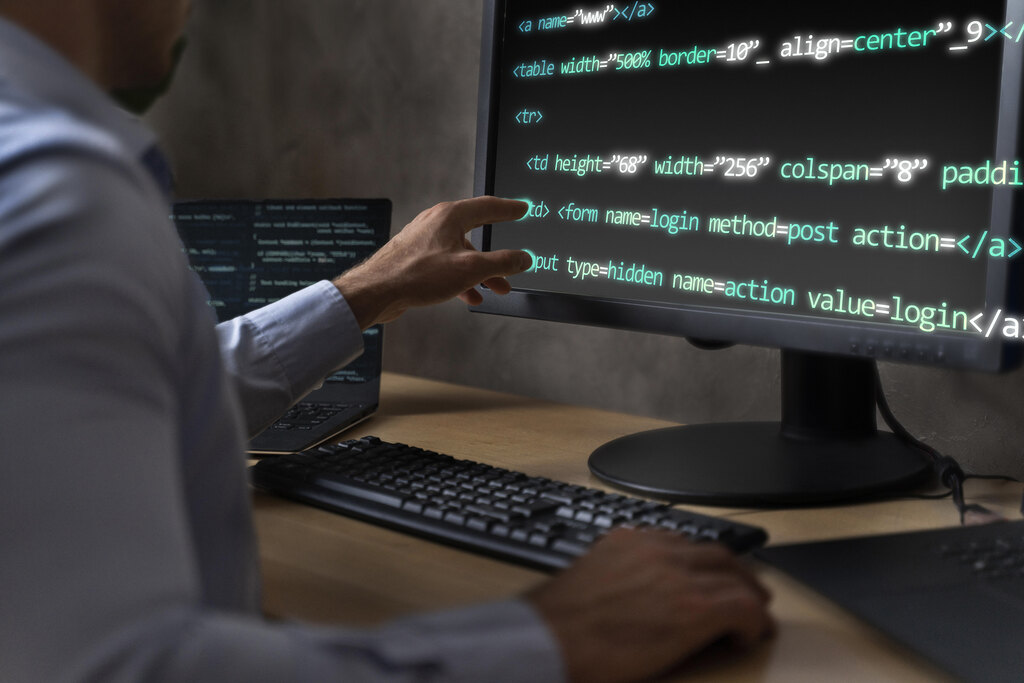
Managing Python os Current Directory
Retrieving Current Directory
To access the current directory in Python, you can use the os.getcwd()
method. This function returns a string representing the current working directory.
Changing Directory
To switch to a different directory, utilize the os.chdir()
method. This function takes the path of the directory you want to navigate to as an argument.
Listing Directory Contents
You can list all the files and directories within the current directory using the os.listdir()
method. This function returns a list containing the names of all entries in the directory.
Checking if a Path is a Directory
If you need to verify whether a given path is a directory or not, you can use the os.path.isdir()
method. It returns True
if the path is a directory and False
otherwise.
Checking if a Path is a File
Similarly, to check if a specific path points to a file, you can employ the os.path.isfile()
method. It returns True
if the path is a file and False
otherwise.
Final Remarks
Understanding how to access and manipulate the current directory is crucial for efficient file handling and organization in your Python projects. By mastering methods like os.getcwd()
and Path.cwd()
, you can streamline your workflow and avoid errors when dealing with file paths.
Now that you’re equipped with the knowledge to navigate and manage the current directory in Python, put these techniques into practice in your coding endeavors. Experiment with different directory operations, explore additional functionalities offered by the os
module, and enhance your Python skills. Embrace the power of controlling directories effortlessly to boost your productivity and efficiency in programming tasks.
Frequently Asked Questions
How to Change the Current Working Directory?
To change the current working directory in Python, use the os.chdir()
method. Provide the path of the desired directory as an argument to this method to switch to a new directory.
How to Get and Change the Current Directory in Python?
To get the current directory, use os.getcwd()
method. To change the directory, utilize os.chdir()
method by passing the path of the new directory.
What is the Current Working Directory?
The current working directory is the directory in which your Python script is currently executing. It serves as the reference point for any file operations performed by your script.
What is the Python os module?
The os
module in Python provides a way to interact with the operating system. It offers functions to manage files and directories, work with processes, and perform various system-related tasks in a platform-independent manner.
How to Get The Current Directory Using the os.getcwd() Method in Python?
By calling os.getcwd()
method in Python, you can retrieve the absolute path of the current working directory where your script is running from.
What is Path.cwd() Method for Directory?
Path.cwd()
method is used for obtaining the current working directory in Python when using pathlib module. It returns a Path object representing the current directory, providing an alternative approach compared to using os.getcwd()
.
Leave a Reply